Build a Beautifully Animated Login Page using HTML, CSS, and JavaScript
If you want to make a Registration Form that works well on different devices and is easy to create with simple HTML, CSS, and JavaScript, you’re in the right place.
In this article, we’ll show you how to make a Responsive Registration Form using basic HTML, CSS, and JavaScript. Even if you’re just starting out and only know a little about HTML and CSS, you can still make this Registration Form.
A Registration Form is a part of a website or app where users fill in their information, like their name, email, and password.
Take a look at the preview of the Registration Form we’re going to make in this article. I’ve included important input fields that every Registration Form should have.
If you want to create your Login and Sign-up Form using HTML, CSS, and JS, I’ve made a Responsive Registration Form for you. Follow the steps I’ve provided below.
Build a nice Animated Login and Sign-up page
To make a beautifully Animated Login and Sign-up page with HTML, CSS, and JavaScript, follow these steps one by one:
- Create a folder. You can name it whatever you like. Inside this folder, make the following files:
- Create an index.html file. The file name must be “index” with the extension “.html“.
- Create a style.css file. The file name must be “style” with the extension “.css“.
- Create a script.js file. The file name must be “script” with the extension “.js“.
- Once you’ve made these files, copy and paste the provided codes into the specified files. If you don’t want to do this manually, scroll down to the end of this page and click the Download button to get the source code.
- Start by pasting the HTML code into your index.html file.
HTML CODE:
Copy and paste the following HTML code into your index.html file.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.4.2/css/all.min.css">
<script src="https://kit.fontawesome.com/2efc16a506.js" crossorigin="anonymous"></script>
<link rel="stylesheet" href="style.css">
<title>Login Form | pbc-webdev</title>
</head>
<body>
<div class="container" id="container">
<div class="form-container sign-up">
<form>
<h1>Register With</h1>
<div class="social-icons">
<a href="#" class="icon"><i class="fa-brands fa-google-plus-g"></i></a>
<a href="#" class="icon"><i class="fa-brands fa-facebook-f"></i></a>
<a href="#" class="icon"><i class="fa-brands fa-github"></i></a>
<a href="#" class="icon"><i class="fa-brands fa-linkedin-in"></i></a>
</div>
<hr>
<h1>OR</h1>
<hr>
<span>Fill Out The Following Info For Registeration</span>
<input type="text" placeholder="Name" required>
<input type="email" placeholder="Email" required>
<input type="password" placeholder="Password" required>
<button>Sign Up</button>
</form>
</div>
<div class="form-container sign-in">
<form>
<h1>Login With</h1>
<div class="social-icons">
<a href="#" class="icon"><i class="fa-brands fa-google-plus-g"></i></a>
<a href="#" class="icon"><i class="fa-brands fa-facebook-f"></i></a>
<a href="#" class="icon"><i class="fa-brands fa-github"></i></a>
<a href="#" class="icon"><i class="fa-brands fa-linkedin-in"></i></a>
</div>
<hr>
<h1>OR</h1>
<hr>
<span>Login With Your Email & Password</span>
<input type="email" placeholder="Email" required>
<input type="password" placeholder="Password" required>
<button>Login</button>
</form>
</div>
<div class="toggle-container">
<div class="toggle">
<div class="toggle-panel toggle-left">
<h1>Welcome Back!</h1>
<p>Provide your personal details to use all features</p>
<button class="hidden" id="login">Sign In</button>
</div>
<div class="toggle-panel toggle-right">
<h1>Hello</h1>
<p>Register to use all features in our site</p>
<button class="hidden" id="register">Sign Up</button>
</div>
</div>
</div>
</div>
<script src="script.js"></script>
</body>
</html>
CSS CODE:
Next, paste the CSS code into your style.css file.
@import url("https://fonts.googleapis.com/css2?family=Montserrat:wght@300;400;500;600;700&display=swap");
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: "Montserrat", sans-serif;
}
body {
background-color: #004754;
background: linear-gradient(to right, #004754, #bebd00);
display: flex;
align-items: center;
justify-content: center;
flex-direction: column;
height: 100vh;
}
.container {
background-color: #fff;
border-radius: 30px;
box-shadow: 0 5px 15px rgba(60, 48, 48, 0.35);
position: relative;
overflow: hidden;
width: 768px;
max-width: 100%;
min-height: 480px;
}
.container p {
font-size: 14px;
line-height: 20px;
letter-spacing: 0.3px;
margin: 20px 0;
}
.fa-brands {
color: #004754;
}
.container span {
font-size: 12px;
}
.container a {
color: #333333;
font-size: 13px;
text-decoration: none;
margin: 15px 0 10px;
}
.container button {
background-color: #bebd00;
color: #ffffff;
font-size: 12px;
padding: 10px 45px;
border: 1px solid transparent;
border-radius: 0px;
font-weight: 600;
letter-spacing: 0.5px;
text-transform: uppercase;
margin-top: 10px;
cursor: pointer;
}
.container button.hidden {
background-color: transparent;
border-color: #ffffff;
}
.container form {
background-color: #ffffff;
display: flex;
align-items: center;
justify-content: center;
flex-direction: column;
padding: 0 40px;
height: 100%;
}
.container input {
background-color: #cccccc;
border: none;
margin: 8px 0;
padding: 10px 15px;
font-size: 13px;
border-radius: 0px;
width: 100%;
outline: none;
}
.form-container {
position: absolute;
top: 0;
height: 100%;
transition: all 0.6s ease-in-out;
}
.sign-in {
left: 0;
width: 50%;
z-index: 2;
}
.container.active .sign-in {
transform: translateX(100%);
}
.sign-up {
left: 0;
width: 50%;
opacity: 0;
z-index: 1;
}
.container.active .sign-up {
transform: translateX(100%);
opacity: 1;
z-index: 5;
animation: move 0.6s;
}
@keyframes move {
0%,
49.99% {
opacity: 0;
z-index: 1;
}
50%,
100% {
opacity: 1;
z-index: 5;
}
}
.social-icons {
margin: 20px 0;
}
.social-icons a {
border: 1px solid #004754;
border-radius: 25%;
display: inline-flex;
justify-content: center;
align-items: center;
margin: 0 3px;
width: 40px;
height: 40px;
}
.toggle-container {
position: absolute;
top: 0;
left: 50%;
width: 50%;
height: 100%;
overflow: hidden;
transition: all 0.6s ease-in-out;
border-radius: 150px 0 0 100px;
z-index: 1000;
}
.container.active .toggle-container {
transform: translateX(-100%);
border-radius: 0 150px 100px 0;
}
.toggle {
background-color: #004754;
height: 100%;
background: linear-gradient(to right, #004754, #004754);
color: #ffffff;
position: relative;
left: -100%;
height: 100%;
width: 200%;
transform: translateX(0);
transition: all 0.6s ease-in-out;
}
.container.active .toggle {
transform: translateX(50%);
}
.toggle-panel {
position: absolute;
width: 50%;
height: 100%;
display: flex;
align-items: center;
justify-content: center;
flex-direction: column;
padding: 0 30px;
text-align: center;
top: 0;
transform: translateX(0);
transition: all 0.6s ease-in-out;
}
.toggle-left {
transform: translateX(-200%);
}
.container.active .toggle-left {
transform: translateX(0);
}
.toggle-right {
right: 0;
transform: translateX(0);
}
.container.active .toggle-right {
transform: translateX(200%);
}
JAVASCRIPT CODE:
Finally, copy and paste the JavaScript code into your script.js file.
const container = document.getElementById('container');
const registerBtn = document.getElementById('register');
const loginBtn = document.getElementById('login');
registerBtn.addEventListener('click', () => {
container.classList.add("active");
});
loginBtn.addEventListener('click', () => {
container.classList.remove("active");
});
Once you’ve pasted all the codes, open your index.html file in your preferred web browser. You’ll see a responsive, beautiful, animated login and sign-up form.
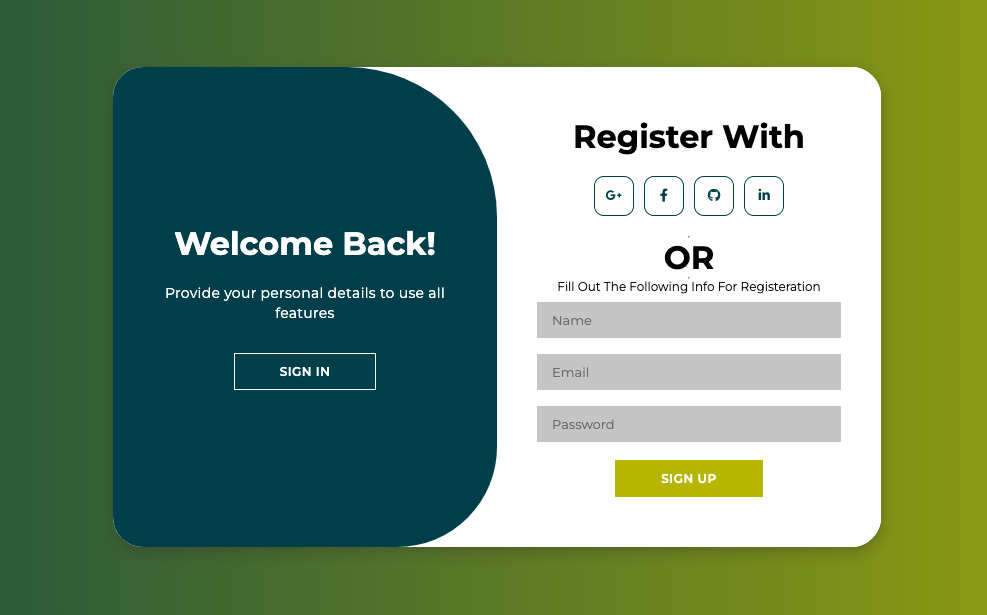
If you encounter any problems while making your Registration Form or if your code isn’t working as expected, you can download the source code files for this Animated Login Form for free by clicking on the Download button.