Easy Way to Make Login and Sign-Up Forms for Websites
Making forms for people to log in or sign up is something developers do a lot. It’s super important for loads of websites. When you learn how to make these forms, you get better at designing and building stuff as a developer.
A login form is a kind of form where users put in their details like a username and password to get into a safe part of a website. Then there’s the registration form, also called a sign-up form, where users create an account on a website or app.
This blog will teach you how to make a flexible Login & Registration Form using HTML, CSS, and JavaScript. It’ll start from the basics of making these forms in HTML, then move on to making them look good with CSS, and finally, add some cool stuff with JavaScript.
This blog will guide you in creating a form similar to the one demonstrated in this video clip.
Making a Login & Sign-Up Form
Step 1:
First, we need to create three files: index.html, styles.css, and main.js. Each file has a job: HTML for structure, CSS for looks, and JavaScript for making things work. Please create a folder on your computer and give it an appropriate name. Inside this folder create the above-mentioned 3 files.
Step 2:
In the index.html file, add the below provided HTML code. This code creates the basic structure of your form.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="styles.css" />
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<title>Build by pbc-WebDev</title>
</head>
<body>
<div class="login-page">
<div class="form">
<form class="register-form">
<input type="text" placeholder="User Name"required />
<input type="password" placeholder="Password" required/>
<input type="text" placeholder="Email ID" required/>
<button>Create</button>
<p class="message">Already Registered? <a href="#">Login</a></p>
</form>
<form class="login-form">
<input type="text" placeholder="User Name" required>
<input type="password" placeholder="Password" required>
<button>Login</button>
<p class="message">Not Registered? <a href="#">Register</a></p>
</form>
</div>
</div>
<script src="main.js"></script>
</body>
</html>
Step 3:
Then, in the styles.css file, add the provided CSS code. You can change the colors, backgrounds, fonts, and sizes if you like. Make it yours!
body {
height: 100vh;
background-size: cover;
background-position: center;
background-color: #333333;
}
.login-page {
width: 360px;
padding: 10% 0 0;
margin: auto;
}
.login-page .form {
position: relative;
z-index: 1;
background: #004754;
max-width: 360px;
margin: 0 auto 100px;
padding: 45px;
text-align: center;
}
.login-page .form input {
font-family: sans-serif;
outline: 1;
background: #f2f2f2;
width: 100%;
border: 0;
margin: 0 0 15px;
padding: 15px;
box-sizing: border-box;
font-size: 16px;
}
.login-page .form button {
font-family: sans-serif;
text-transform: uppercase;
outline: 0;
background: #bebd00;
width: 100%;
border: 0;
padding: 15px;
color: #FFFFFF;
font-size: 14px;
cursor: pointer;
}
.login-page .form button:hover, .login-page .form button:active {
background: #bebd00;
}
.login-page .form .message {
font-family: sans-serif;
margin: 15px 0 15px;
color: aliceblue;
font-size: 14px;
}
.login-page .form .message a {
color: #bebd00;
text-decoration: none;
}
.login-page .register-form {
display: none;
}
Now, when you open your HTML file in any browser, you’ll see your beautiful form as shown in the following picture. But, clicking on the ‘Register‘ link won’t do anything yet.
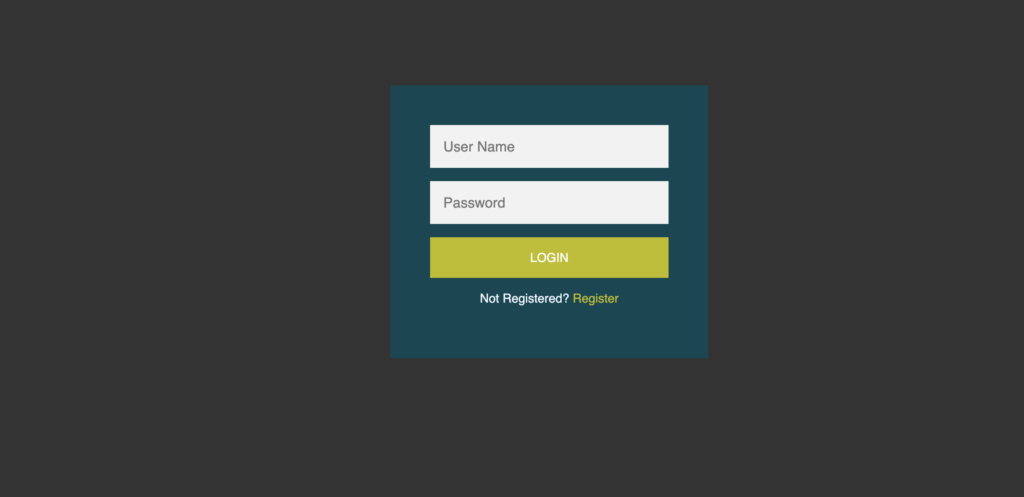
Step 4:
To fix that, you need JavaScript code. Add the provided JavaScript code in your main.js file, refresh your browser, and try clicking on ‘Register‘ again. Now, It should work just like as shown in the above demo video clip.
Conclusion:
Congratulations! You’ve created a login and sign-up form. We are adding more and more forms to explore on this website to boost your form-making skills. If you found this helpful, consider sharing it with others. Your support keeps us making more helpful content for developers. Thank you!
If you encounter any issues or if your form isn’t working as planned, no worries! You can download the source code for free by clicking the following Download button. This will help you out if you’re stuck.